Setting up the Tailwind CSS framework
Discover how to configure this popular utility-first framework so you too can start using it!
Warning
Since this article was published, Tailwind 1.0 has been released. As such, some parts of this article are no longer up to date.
If you have never heard of Tailwind, you might be forgiven for wondering what all the fuss around this new CSS framework is about. Unlike Bootstrap, Foundation, Bulma and other well known CSS frameworks, Tailwind is not a UI Kit. It doesn’t have a long list of components and pre-made templates that you can use to quickly build out a website.
Instead, it’s a library of low-level classes that is designed to let you rapidly build a website without having to fight with the default styles that Bootstrap et al impose on you.
Unlike Bootstrap, Foundation and other CSS frameworks, Tailwind doesn’t use semantic class names – e.g. card
, banner
etc. Instead, it uses what are known as utility classes (or sometimes
functional or atomic CSS). These classes describe the style, rather than
the component.
Instead of trying to rewrite the excellent description of Tailwind its creator Adam Watham has already written, I’d suggest popping over to the Tailwind docs and reading the What is Tailwind page to find out more.
Before we get started
So as with any tutorial, there are going to be a couple of things that I do differently to you. Don’t feel like you need to follow along exactly. I’d strongly recommend doing things the way that you would normally do them.
I use Laravel Valet to power my local development server. Feel free to use whatever tool you normally use. Try to minimise the amount of new stuff you’re learning, and the focus of this tutorial – how to set up Tailwind – will be easier to retain.
Required knowledge
I’d rather be upfront with you about the things you need to know before progressing. In order to follow along with this tutorial, you will need to have either NPM or Yarn installed, and have a basic understanding of how they work. I’ll be giving you the commands you need to run, but knowing how they work will make this tutorial a lot easier to follow.
Let's do this
1. Create a folder
The first thing we need to do is create a project. If you’re using a tool like MAMP, WAMP or XAMPP, these tools will handle this bit for you. Otherwise, if you’re using something like Valet, open up your terminal.
Navigate to the folder where your web projects live – in my case that’s code>~/Sites. Once you’re there, create a new folder (you could do this via Explorer or Finder if you’re more comfortable with this). For the purpose of this tutorial, let’s call it tailwinddemo.
Once
the project folder has been created, we’ll open it up in a code editor.
There’s a number of ways you could do this – drag the folder onto the
code editor shortcut or link in the toolbar, open the code editor and
open the folder, or do it via the command line. For the latter, in the
terminal type cd tailwinddemo
. Then open your code editor of choice – in my case, it’s VSCode. To do this, type code .
. For Sublime Text (if you have the shortcut set up), you’d type sublime .
etc.
2. Create an index.html file
Now that the project is open in a code editor, we’re going to create an index.html
file. (We’ll keep this as basic as possible so it’s easier for you to translate it to your tool of choice).
Open the file and add some skeleton code. If you use Emmet like me, you can type html:5
and tab to auto expand. If you aren’t using Emmet, you can write copy
the below or use a boilerplate like HTML5 Boilerplate instead.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
</body>
</html>
In the <head>
section, after the <title>
, we’ll create a link to the stylesheet we’ll be generating. This will be in /css/app.css
. You can call this file whatever you’d like – style.css, main.css, hell even css.css – I just personally prefer app.
To help things make sense, we’ll replicate Bootstrap’s jumbotron component. Let’s add the required markup and place it between the body tags:
<section>
<div>
<h1>Hello, world!</h1>
<p>This is a simple hero unit, a simple jumbotron-style component for calling extra attention to featured content or information.</p>
<hr>
<p>It uses utility classes for typography and spacing to space content out within the larger container.</p>
<button>Learn more</button>
</div>
</section>
One quick note: I’ve used button instead of anchor
tags. You should only use an anchor tag if you’re linking somewhere. If
you want a button – as is suggested in the Jumbotron demo by its use of role=button
– use a button tag instead.
We won’t add any classes to it just yet. We’ll save that for part 2. We just want to make sure everything’s working. Your browser should look the same as the screenshot below.
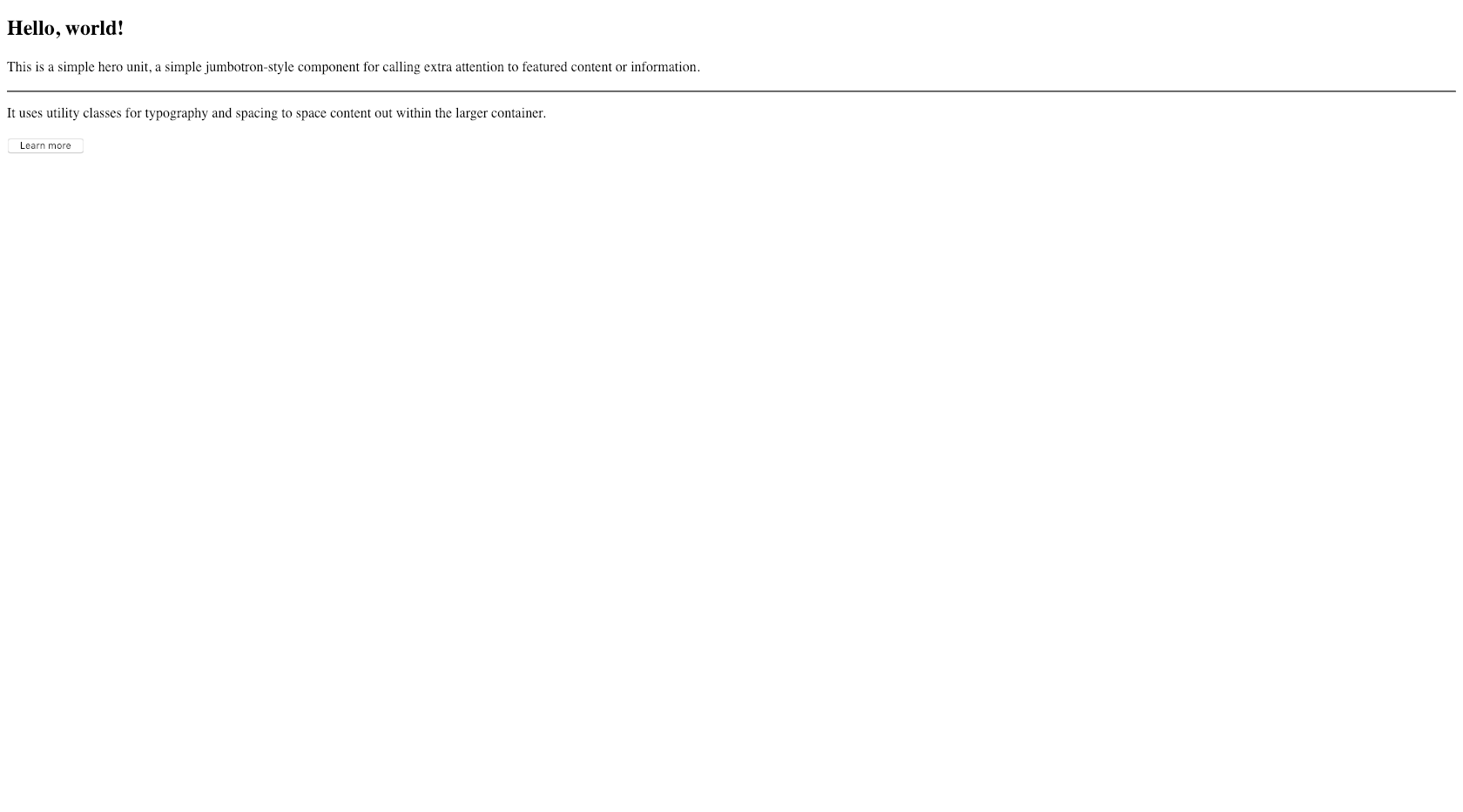
3. Set up our package manager
Now we’ve got the base markup set up, let’s get to the nuts and bolts of this tutorial – how to setup and configure Tailwind.
As
I mentioned before, you’ll need to have NPM or Yarn set up before you
go any further. For the purpose of this tutorial, I’ll be using Yarn,
which again is my personal preference and not because it’s necessarily
better. All of the commands are interchangeable – yarn add package-name -D
is the same as npm i package-name --save-dev
.
You also need a build tool to use Tailwind. In this tutorial, I’ll be using Laravel Mix. If you’ve never used it before, it’s a tool that has emerged out of the Laravel community and is built upon Webpack. It has a really simple to use API compared to Gulp, Grunt and vanilla Webpack. Also, don’t be worried about it being called Laravel Mix; you don’t need to be working with Laravel to use it.
If you want me to create tutorials specific to those other build tools, let me know in the comments and I’ll write them up.
Steps
1) First things first, we need to create a package.json
file. To do this, we’ll open up our terminal window and run yarn init
. This will then ask a series of questions the default answers are fine so feel free to hit enter on all of them.
2)
Next, we need to add some scripts to the package.json file. These will
make running Laravel Mix easier. Open the file and then paste in the
following on a new line within the {}
.
"scripts": {
"dev": "yarn run development",
"development": "NODE_ENV=development node_modules/webpack/bin/webpack.js --progress --hide-modules --config=node_modules/laravel-mix/setup/webpack.config.js",
"watch": "yarn run development --watch",
"prod": "yarn run production",
"production": "NODE_ENV=production node_modules/webpack/bin/webpack.js --no-progress --hide-modules --config=node_modules/laravel-mix/setup/webpack.config.js"
},
3) Next, we need to install Tailwind. In the terminal window, run yarn add tailwindcss -D
.
We’re installing it as a dev dependency because there’s no real need to
have it running on the server. If you have a reason, feel free to omit
the -D so it’ll install as a normal dependency.
4) Once we’ve
installed Tailwind, we need to create a config file. This is where all
of the settings are stored. To do this in your terminal window, type ./node_modules/.bin/tailwind init
. This will output a file called tailwind.js
in the root of the project. If like me, you prefer to have all your
config files follow the same naming convention, you can pass in an
additional argument, and it’ll create the config file with that name
(e.g. .node_modules/.bin/tailwind init tailwind.config.js
.
5) Now that Tailwind is installed and initialised, we need to install our build tool. To install Mix, in the terminal run yarn add laravel-mix -D
. Again, if you want to run the build tool on the server, feel free to omit the -D at the end. (As a quick note, Laravel Mix can take a while to download so just be patient).
4. Setting up the stylesheet
Next, we need to create a source file where we will define our Tailwind imports and any other custom classes we want to add. For the purposes of this demo, we’ll use PostCSS. I’ll be adding tutorials for how to do this in Sass and Less in the next couple of weeks.
It’s
entirely up to you how you want to structure this. For the sake of
keeping it simple, we’ll call the folder that will contain our source
CSS file src
and create another folder in it called css
. Within the css
folder, we’ll create a file called app.css
. This will allow us to create another folder called js
if we want, and add all our custom JavaScript in there.
Once you’ve done that, open the app.css
file in src/css/
and add the following:
@tailwind preflight;
@tailwind components;
@tailwind utilities;
These three lines are all you need to configure Tailwind. The example stylesheet in the Tailwind docs is pretty much the same, just with comments that explain more about the reasoning behind the order, and some additional options.
5. Setting up Laravel Mix
Once Laravel Mix has downloaded, in the root of your folder create a new file called webpack.mix.js
and then open it. As previously mentioned, we’ll be using PostCSS for
this tutorial. Don’t worry if you’ve never used it before and are more
used to Sass or Less; it’s pretty similar.
In the file, add the following code:
const mix = require('laravel-mix');
const tailwindcss = require('tailwindcss');
mix.postCss('src/css/app.css', 'css/', [
tailwindcss('./tailwind.config.js'),
]);
Save the file and then in your terminal window, run yarn dev
. You should see a notice that the process is done and it was successfully compiled.
If you check your code editor, you should now see a new folder and file have been created – css/app.css
. This file has all the compiled code. If you switch to your browser, you’ll now see we’re getting the styles applied by normalize.css
, which is included in Tailwind.
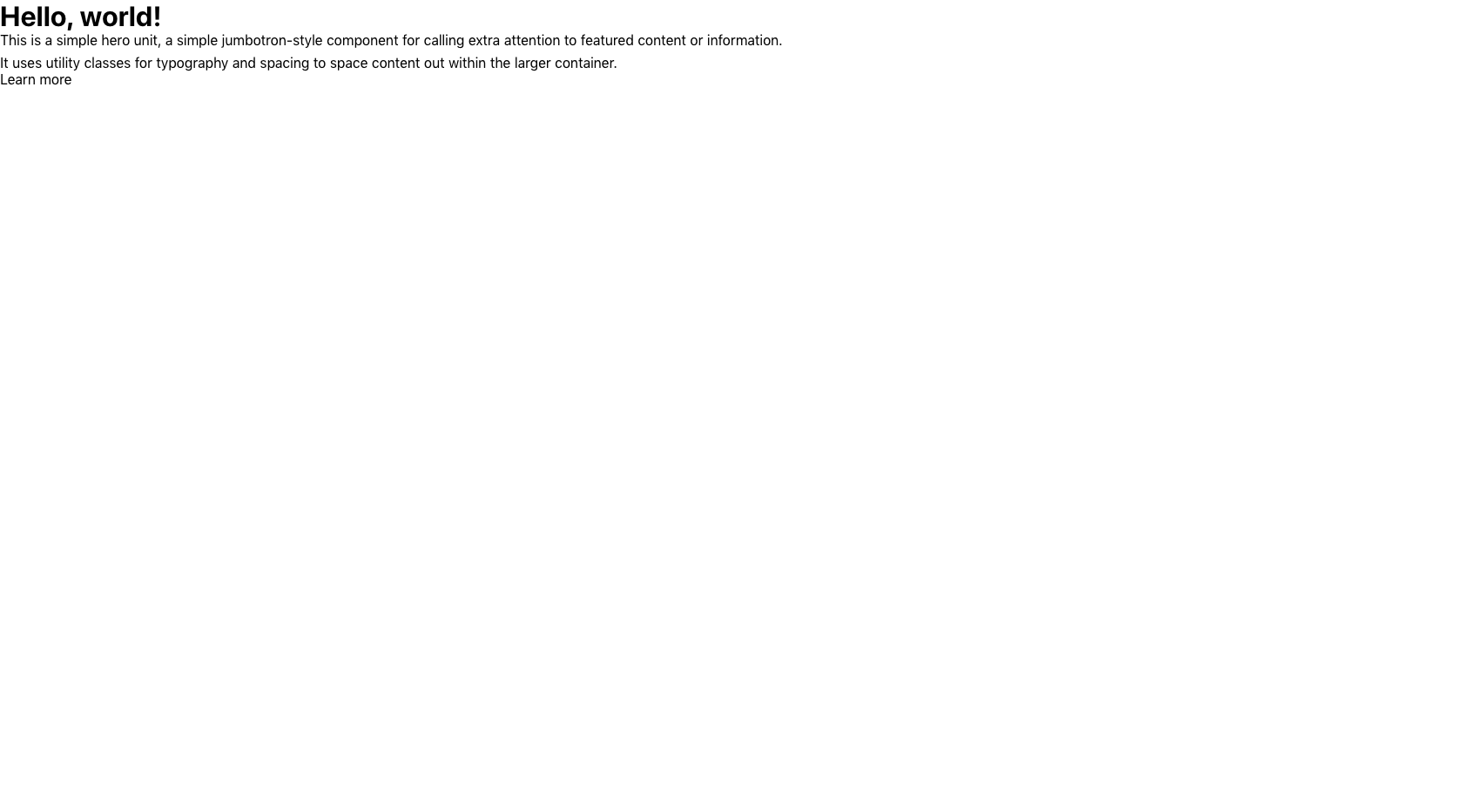
More about the commands
yarn dev
is one of the commands defined in the scripts section we posted into package.json earlier. The others are yarn watch
and yarn prod
. The other two are the main commands, and can be accessed easily using the shorter versions (dev/prod).
The key difference between yarn dev
and yarn prod
is that dev will compile everything un-minified, while prod will minify everything.
Wrapping up
We now have a working install of Tailwind CSS. Tomorrow, I’ll look at putting together a video walk through. I’ll also put together a walk through of replicating Bootstrap’s Jumbotron – which we’ve added the markup for. In the meantime, if anything is unclear or you have any questions, let me know below and I’ll try my best to answer them.